作者:matrix
发布时间:2022 年 1 月 7 日
分类:Python
之前是本地Python环境安装Django项目直接运行和断点,现在尝试切换到vscode~
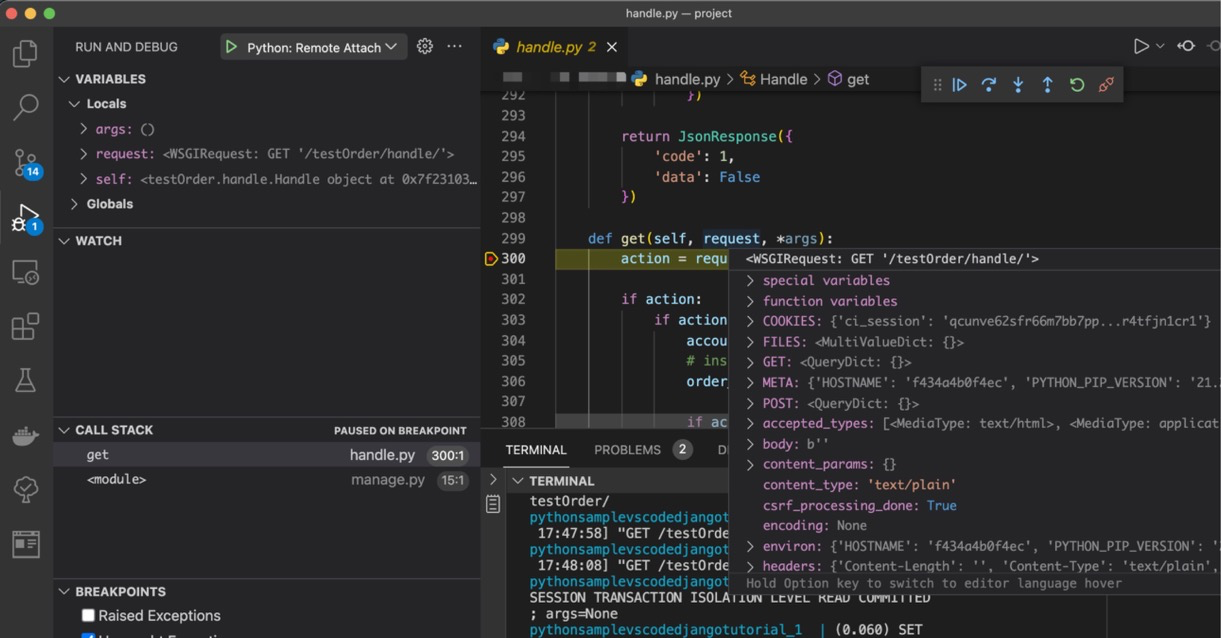
vscode插件
https://marketplace.visualstudio.com/items?itemName=ms-vscode-remote.remote-containers
https://marketplace.visualstudio.com/items?itemName=ms-python.python
Dockerfile配置
用于创建docker镜像
项目根目录中创建Dockerfile文件
# syntax=docker/dockerfile:1
FROM python:3.10
#设置容器中环境变量
ENV RUN_ENV='develop'
WORKDIR /data
COPY requirements.txt .
RUN pip install -i https://pypi.douban.com/simple/ -r requirements.txt
# 方式2.安装依赖
# RUN pip install -i https://pypi.douban.com/simple/ django pytz pymysql mysqlclient requests numpy python-dateutil matplotlib pandas_datareader scipy sklearn
根目录中需要有requirements.txt
docker-compose配置
用于开启容器,挂载开发目录以及端口
项目根目录中创建docker-compose.debug.yml文件
version: '3.4'
services:
pythonsamplevscodedjangotutorial:
image: django-dev:1.1
build:
context: .
dockerfile: ./Dockerfile
volumes:
- ./:/data
# command: ["sh", "-c", "pip install -i https://pypi.douban.com/simple/ debugpy -t /tmp && python /tmp/debugpy --wait-for-client --listen 0.0.0.0:5678 /data/manage.py runserver 0.0.0.0:8000 --nothreading --noreload"]
command: ["sh", "-c", "python /tmp/debugpy --listen 0.0.0.0:5678 /data/manage.py runserver 0.0.0.0:8000 --nothreading --noreload "]
ports:
- 8000:8000
- 5678:5678
说明:
volumes表示 映射本地路径./
-->容器路径/data
5678为断点监听端口
8000为项目访问端口
配置debug
配置vscode debug
项目根目录中编辑或创建.vscode/launch.json
{
"configurations": [
{
"name": "Python: Remote Attach",
"type": "python",
"request": "attach",
"port": 5678,
"host": "localhost",
"pathMappings": [
{
"localRoot": "${workspaceFolder}",
"remoteRoot": "/data"
}
]
}
]
}
启动容器
$ docker-compose -f ./docker-compose.debug.yml up
添加断点后,浏览器访问127.0.0.1:8000即可调试
参考:
https://code.visualstudio.com/docs/containers/docker-compose#_python
https://docs.docker.com/compose/compose-file/compose-file-v3/#build
https://www.youtube.com/watch?v=x7lZAmMVo2M
作者:matrix
发布时间:2021 年 2 月 8 日
分类:零零星星
本地用miniconda创建的python环境,程序的打包和运行都是正常。但是搬到了其他电脑运行就是失败,死活报错Failed to execute script pyi_rth_certifi
。尝试其他打包参数-p --datas --hidden-import,更换python版本和Pyinstaller的develop版本也一样。
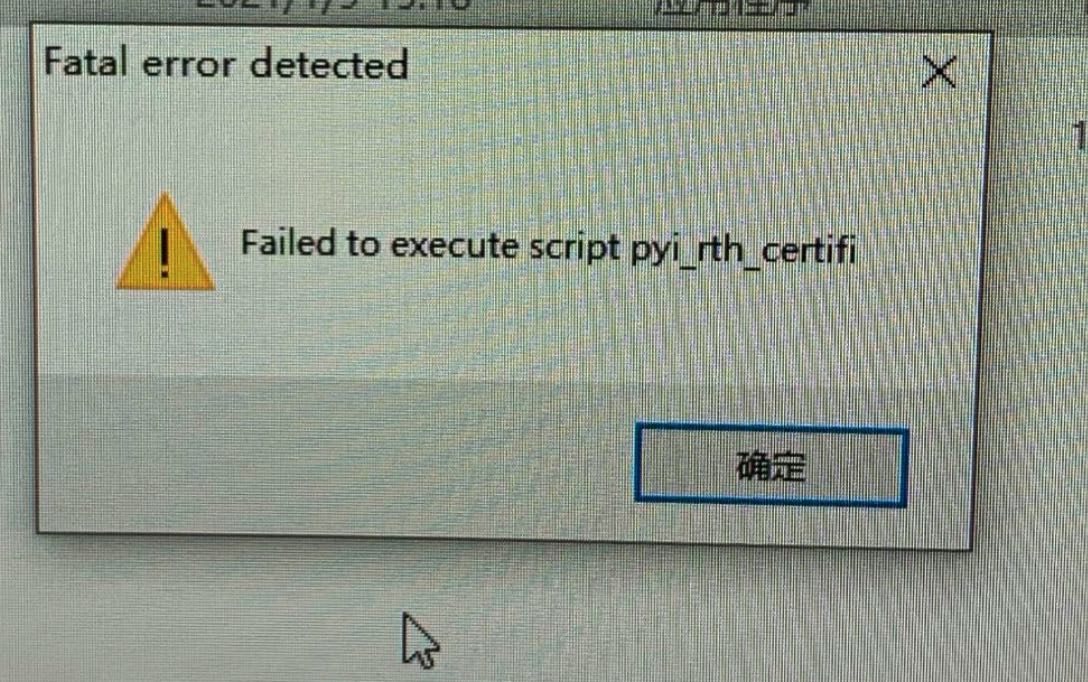
最后还是在cmd命令行打开调试才显示具体信息。
删除dist/,build/
目录再使用-c
参数重新打包,之后从cmd执行程序才看到详细提示。
最终并不是依赖的问题,只是因为运行环境缺少dll文件导致。😂
解决办法
安装OpenSSL库
下载页面:https://slproweb.com/products/Win32OpenSSL.html
默认安装之后就可以了
参考:
https://bugs.python.org/issue39344
https://slproweb.com/products/Win32OpenSSL.html
Pyinstaller打包报错Failed to execute script pyi_rth_pkgres
https://stackoverflow.com/questions/32093559/exe-file-created-by-Pyinstaller-not-find-self-defined-modules-while-running
https://wiki.openssl.org/index.php/Binaries
作者:matrix
发布时间:2020 年 8 月 31 日
分类:Python 零零星星
第一次drop超过GB的数据表,没想到竟然会执行的这么慢。尝试过TRUNCATE
和DROP
都不满意。
后来就直接找到数据库储存的文件来删除,这样比起使用sql语句操作会快得多,但也是危险操作,无法找回。
删除操作脚本
运行环境 python3.7,依赖pymysql
,根据自身情况配置变量mysql_data_dir,db_config,table_names,condition_save
fast_drop_table.py
#codeing=utf-8
"""
快速清空超大数据表 保留想要数据
"""
import pymysql
import os
mysql_data_dir = '/mnt/mysql_data/db_name/' #数据库文件所在路径
# 数据库连接配置
db_config = {'host': '127.0.0.1', 'port': 3306, 'user': 'user', 'password': 'password', 'db': 'db_name', 'charset': 'utf8'}
# 需要清空操作的数据表
table_names = [
"com_hhtjim_badata_trades_eos_this_quarter",
"com_hhtjim_badata_trades_eth_this_quarter",
]
# 数据表保留的查询条件
condition_save = "timestamp > '2020-02-20T00:00:00Z'"
# condition_save = False# 不保留
class Db:
'''
简单数据库连接操作类
'''
def __init__(self,**kwargs):
self.connection = pymysql.connect(**kwargs)
self.cursor = self.connection.cursor()
if __name__ == "__main__":
mysql = Db(**db_config)
for table_name in table_names:
os.link('{}{}.frm'.format(mysql_data_dir,table_name), '{}{}.frm.h'.format(mysql_data_dir,table_name))
os.link('{}{}.ibd'.format(mysql_data_dir,table_name), '{}{}.ibd.h'.format(mysql_data_dir,table_name))
mysql.cursor.execute('CREATE TABLE {0}_back like {0}'.format(table_name))
mysql.connection.commit()
if condition_save:
mysql.cursor.execute("INSERT INTO {0}_back SELECT * FROM {0} WHERE {1} ;".format(table_name,condition_save))
mysql.connection.commit()
mysql.cursor.execute("drop table {}".format(table_name))
mysql.connection.commit()
mysql.cursor.execute("alter table {0}_back rename to {0};".format(table_name))
mysql.connection.commit()
os.unlink('{}{}.frm.h'.format(mysql_data_dir,table_name))
os.unlink('{}{}.ibd.h'.format(mysql_data_dir,table_name))
print('succeed: {}'.format(table_name))
具体步骤
### 找到frm,ibd文件
根据数据库存储路径找到需要删除的表名的frm,ibd文件。
### 建立硬连接
$ ln mytable.ibd mytable.ibd.h
$ ln mytable.frm mytable.frm.h
### 备份表结构
CREATE TABLE mytable_back like mytable;
### 备份想要保留的数据
INSERT INTO mytable_back SELECT * FROM mytable WHERE timestamp > '2020-02-27T00:00:00Z' ;
### 删除旧表
drop table mytable;
### 修改备份表名字
alter table mytable_back rename to mytable;
### 删除硬连接
$ rm -f mytable.frm.h mytable.ibd.h
参考:
https://blog.csdn.net/weixin_34034261/article/details/86250223
作者:matrix
发布时间:2019 年 11 月 26 日
分类:Python
asyncio
异步操作需要关键字async
,await
。
async
用来声明方法函数,await
用来声明耗时操作。
但是await
关键字后面要求为awaitable对象
且只能在async方法内部使用,不能在外部入口中使用。asyncio的语法其实是系统内部实现了yield from
协程。
aiohttp
用来代替requests的请求库,且支持异步操作。
主要优点体现在并发请求多个耗时任务时,自动安排耗时时的操作,避免cpu等待一个一个请求。
单个请求操作
import aiohttp
import asyncio
#get 请求
async def get():
async with aiohttp.request('GET','https://api.github.com/users/Ho',params={'arg1':123}) as response:
# response.request_info # 请求信息
return await response.json()
rel = asyncio.run(get())
# 或者使用下面方式 手动关闭异步事件循环
# loop = asyncio.get_event_loop()
# rel = loop.run_until_complete(get())
# loop.close()
print(rel)
多个并发请求操作
主要区别在于异步任务的添加操作,运行。
请求测试url:
http://link/await/1 # delay 1sec
http://link/await/2 # delay 2sec
...
请求测试:
import aiohttp
import asyncio
#get 请求
async def get():
async with aiohttp.request('GET','http://link/await/1') as response:
return await response.text()
# 所有请求任务
async def all_req():
#async with asyncio.Semaphore(5): 设置并发的连接数
# https://docs.python.org/zh-cn/3/library/asyncio-sync.html#asyncio.Semaphore
task = []
#添加请求任务
for i in range(5):
task.append(asyncio.create_task(get()))
#create_task 方法等同于 ensure_future()方法
#手册建议首选 create_task方法
# https://docs.python.org/zh-cn/3/library/asyncio-future.html?highlight=ensure_future#asyncio.ensure_future
return await asyncio.gather(*task)#传入参数 tuple类型 作为位置参数
# 等同于 asyncio.gather(get(),get())
# gather()方法用于收集所有任务完成的返回值,如果换成wait()方法会返回任务tuple对象,(done,pending)
rel = asyncio.run(all_req())
print(rel)
# 总共5个请求任务返回:
# 总耗时1秒多,相比同步的5秒+好N多。
"""
['sleep 1 second is done', 'sleep 1 second is done', 'sleep 1 second is done', 'sleep 1 second is done', 'sleep 1 second is done']
[Done] exited with code=0 in 1.955 seconds
"""
tell why??
测试发现Semaphore方法设置的请求并发数量跟本不起作用,nginx的access.log
以及Proxifier
看到的一次性请求量都不是代码中设置的数量。
使用uvloop优化异步操作
uvloop用于提升协程的速度。
uvloop使用很简单,直接设置异步策略就好了。
import asyncio
import uvloop
#声明使用 uvloop 事件循环
asyncio.set_event_loop_policy(uvloop.EventLoopPolicy())
测试遇到很多报错,基本上都是await和async使用的问题。
异步请求的分块chunk并发控制
自行chunk操作
自己按照所有任务的list列表进行chunk切割,然后分块进行请求,每块中固定chunk数量的任务。基本可以实现想要的并发限制操作
async def _bulk_task(num,current_page = 1):
"""批量创建异步任务
"""
task = []
for i in range(num):# 每次10个连接并发进行请求
task.append(asyncio.create_task(get(current_page)))
current_page += 1
return await asyncio.gather(*task)
# 主要进行chunk操作的函数
def run_task(total,chunk,offset_start_page = 1):
"""运行分块处理的批量任务
Arguments:
total int 总请求数
chunk int 每次并发请求数
offset_start_page int 初始分块开始的页数(偏移页数),正常默认为1
Yields:
返回收集的异步任务运行结果
"""
length = math.ceil(total/chunk)
for i in range(length):
start_page = i * chunk + offset_start_page # 当前分块开始的页数
haldle_num = chunk# 当前需要并发处理的数量
#处理结尾的块
if i == length - 1:
# print(':::',chunk,start_page + chunk - offset_start_page)
haldle_num = min(chunk,total + offset_start_page - start_page)
# print('当前分块下标:{},当前分块需要处理的总数:{},当前分块开始页数:{}'.format(i,haldle_num,start_page))
rel = asyncio.run(_bulk_task(haldle_num,start_page))
yield rel
rel = run_task(123,10)# 123总任务 每10条并发请求
for i in rel:
print(i)
独立封装
封装为async_curl类,以后可以直接import使用
https://raw.githubusercontent.com/Hootrix/com.gllue.portal/master/async_curl.py
参考:
https://www.cnblogs.com/Summer-skr--blog/p/11486634.html
https://hubertroy.gitbooks.io/aiohttp-chinese-documentation/content/aiohttp%E6%96%87%E6%A1%A3/ClientUsage.html#%E6%84%89%E5%BF%AB%E5%9C%B0%E7%BB%93%E6%9D%9F
https://docs.Python.org/zh-cn/3/library/asyncio-eventloop.html#asyncio.get_running_loop
https://segmentfault.com/q/1010000008663962
http://www.ruanyifeng.com/blog/2019/11/Python-asyncio.html
https://blog.csdn.net/qq_37144341/article/details/89471603
https://www.jianshu.com/p/8f65e50f39b4
作者:matrix
发布时间:2019 年 10 月 14 日
分类:Python
搜索结果一大堆但都没有找到支持url和local path两种读取方式的操作。
留着便于以后直接使用。
gits: https://gist.github.com/Hootrix/cf3e75b1fa6d3d404bc99787f89687f1
import requests,tempfile, zipfile,os
def read_file_for_zip(zip_url, callback=None):
"""
读取zip包内的文件
:param zip_url:zip路径/url
:param callback:读取操作的回调函数 若函数返回false 则不会读取下一个文件
:return:
"""
with tempfile.TemporaryFile('w+b') as tmpfile: # 生成临时文件
# 判断是否为本地文件
if os.path.isfile(zip_url):
#进行本地复制。没必要
# with open(zip_url,'rb') as f:
# while True:
# chunk = f.read(1024)
# if not chunk:
# break
# tmpfile.write(chunk)
tmpfile = zip_url
else:#进行http请求
r = requests.get(zip_url, stream=True)
for chunk in r.iter_content(chunk_size=1024):
if chunk:
tmpfile.write(chunk)
assert zipfile.is_zipfile(tmpfile), '不是zip文件'
zf = zipfile.ZipFile(tmpfile)
for name in zf.namelist(): # list e.g. ['Brave Browser.url', 'Express VPN.url', 'ssl.txt', 'What is my IP.url']
if callable(callback):
# zf.read(name) #读取
if callback(name, zf) is False:# 函数返回false 会终止下一个文件的读取
break
### 例子
def cb(filename,context):
if filename.endswith('.txt'):
print(context.read(filename).decode('utf-8'))
# print( context.read(filename))
return False #终止下一个文件的读取
read_file_for_zip('https://cdn-01.openload.cc/S9Y7m488n8/22c3c58b-1571037628/ssl_proxies.zip',cb)
具体使用见上面例子
兼容大文件url的下载处理
p.s.
在线压缩包读取:
https://extract.me/cn/
参考:
http://www.liujiangblog.com/course/Python/62
https://docs.python.org/2/library/tempfile.html
作者:matrix
发布时间:2019 年 2 月 27 日
分类:零零星星
TeamViewer使用频繁或者被检测到某些ip段中会被标记为商业行为的使用,也就会要求付费。对应的TeamViewer ID也就会被要求进行商业授权许可。
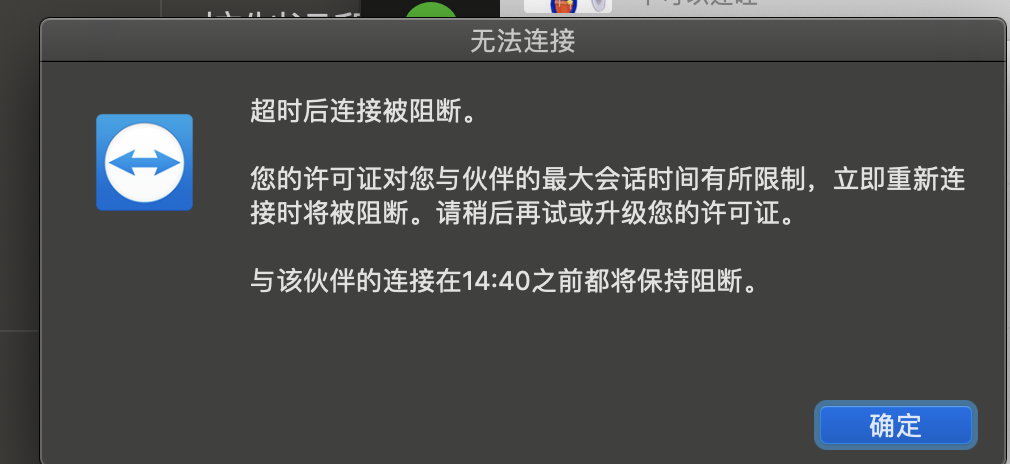
本来之前使用都是正常,今天给朋友远程执行脚本命令,我连接控制了半分钟就提示断开连接「超时后连接被阻断」,然后等待时间过后就提示了监测到「商业行为」。网上说TeamViewer修改了商业行为的判定导致客户大量流失,TeamViewer目前报价对于单用户单连接/年需要¥2500,有点贵啊。期间尝试过mac端的向日葵远程控制端和系统自带的屏幕共享
,前者只能查看不能操作,后者完全连接不上亦或使用内网VNC地址成功,使用apple id就连接失败,mac端qq就根本没这个功能。
方法0
使用其他工具:
https://anydesk.com/zhs
https://sunlogin.oray.com/zh_CN/download
http://www.xt800.cn/download
参考:https://www.appinn.com/alternative-teamviewer/
有人建议使用俄罗斯版本:https://www.teamviewer.com/ru/
方法1
申述TeamViewerID为个人用户使用,最快7天内解决申请。
https://www.teamviewer.com/en/support/commercial-use-suspected/
2019年3月11日 22:36收到邮件“Your TeamViewer ID has been reset to free”
方法2
TeamViewer会把ID进行标记,所以换一个新的ID就可以使用了。
使用虚拟机VirtualBox
来使用TeamViewer,如果被检测有商业行为就给虚拟机重新初始化MAC地址。这样就可以切换新ID
方法3
使用脚本修改,切换TeamViewer新ID
感谢@zhovner的一键切换脚本TeamViewer ID Changer for MAC OS解决帮了大忙:
测试版本:TeamViewer for macOs V14.0.13880版本
python 2.7.10测试运行
#!/usr/bin/env python
#coding:utf-8
import sys
import os
import glob
import platform
import re
import random
import string
print('''
--------------------------------
TeamViewer ID Changer for MAC OS
--------------------------------
''')
if platform.system() != 'Darwin':
print('This script can be run only on MAC OS.')
sys.exit();
if os.geteuid() != 0:
print('This script must be run form root.')
sys.exit();
if os.environ.has_key('SUDO_USER'):
USERNAME = os.environ['SUDO_USER']
if USERNAME == 'root':
print('Can not find user name. Run this script via sudo from regular user')
sys.exit();
else:
print('Can not find user name. Run this script via sudo from regular user')
sys.exit();
HOMEDIRLIB = '/Users/' + USERNAME + '/library/preferences/'
GLOBALLIB = '/library/preferences/'
CONFIGS = []
# Find config files
def listdir_fullpath(d):
return [os.path.join(d, f) for f in os.listdir(d)]
for file in listdir_fullpath(HOMEDIRLIB):
if 'teamviewer'.lower() in file.lower():
CONFIGS.append(file)
if not CONFIGS:
print ('''
There is no TemViewer configs found.
Maybe you have deleted it manualy or never run TeamViewer after installation.
Nothing to delete.
''')
# Delete config files
else:
print("Configs found:\n")
for file in CONFIGS:
print file
print('''
This files will be DELETED permanently.
All TeamViewer settings will be lost
''')
raw_input("Press Enter to continue or CTR+C to abort...")
for file in CONFIGS:
try:
os.remove(file)
except:
print("Cannot delete config files. Permission denied?")
sys.exit();
print("Done.")
# Find binaryes
TMBINARYES = [
'/Applications/TeamViewer.app/Contents/MacOS/TeamViewer',
'/Applications/TeamViewer.app/Contents/MacOS/TeamViewer_Service',
'/Applications/TeamViewer.app/Contents/Helpers/TeamViewer_Desktop',
]
for file in TMBINARYES:
if os.path.exists(file):
pass
else:
print("File not found: " + file)
print ("Install TeamViewer correctly")
sys.exit();
# Patch files
def idpatch(fpath,platf,serial):
file = open(fpath, 'r+b')
binary = file.read()
PlatformPattern = "IOPlatformExpert.{6}"
SerialPattern = "IOPlatformSerialNumber%s%s%sUUID"
binary = re.sub(PlatformPattern, platf, binary)
binary = re.sub(SerialPattern % (chr(0), "[0-9a-zA-Z]{8,8}", chr(0)), SerialPattern%(chr(0), serial, chr(0)), binary)
file = open(fpath,'wb').write(binary)
return True
def random_generator(size=8, chars=string.ascii_uppercase + string.digits):
return ''.join(random.choice(chars) for _ in range(size))
RANDOMSERIAL = random_generator()
RANDOMPLATFORM = "IOPlatformExpert" + random_generator(6)
for file in TMBINARYES:
try:
idpatch(file,RANDOMPLATFORM,RANDOMSERIAL)
except:
print "Error: can not patch file " + file
print "Wrong version?"
sys.exit();
print "PlatformDevice: " + RANDOMPLATFORM
print "PlatformSerial: " + RANDOMSERIAL
print('''
ID changed sucessfully.
!!! Logout User Or Restart computer before using TeamViewer !!!!
''')
脚本执行成功会显示ID changed sucessfully.
之后重启电脑或者注销用户启用就好了。之后打开teamViewer会发现是新的ID。
主要兼容11,12,和14.0版本
14.0.13880 mac版本:https://www.malavida.com/en/soft/teamviewer/mac/
14.0.13488 windows:https://www.filepuma.com/download/teamviewer_14.0.13488-20699/
12.0.72647 mac版本:https://teamviewer.en.uptodown.com/mac/download/1510547
P.S. 4月份发现脚本无法切换ID
,软件会提示商业使用,但是使用上依然没问题
PEACE~
参考:
https://gist.github.com/zhovner/b1d72f3465c46e7b58a4ea42d625c3e8
https://community.spiceworks.com/topic/1151930-teamviewer-5-minute-timelimit
作者:matrix
发布时间:2014 年 9 月 1 日
分类:Python
初玩Python很不习惯那个md5函数。还好有人分享了相关代码,非常感谢。
import hashlib
def md5 (s, raw_output = False):
res = hashlib.md5 (s)
if raw_output:
return res.digest ()
return res.hexdigest ()
如果是Python2.5 :
# Python 2.5+
import hashlib
hashlib.md5("welcome").hexdigest()
# pre-2.5, removed in Python 3
import md5
md5.md5("welcome").hexdigest()
参考:Python 实现PHP内置MD5函数方法